From React to React Hooks
This blog post gives an overview of React, React's history, why we use it, and how we can pivot from using classic class component to React Hooks. Also, a simple demonstration video is included at the end so you can follow along.
Introdcution
In the world of software development, there will be one phrase that you would hear every day - seperation of concerns. Everything is built on top of layers of abstration which is a way of seperation of concerns.
From HTML to JavaScript
Now let us think about the history of web applications. In the beginning, we just want to achieve one simple task - to display data or information on the Internet. Therefore, there is the birth of HTML and XML which browser can use to build a DOM tree and render. However, what is the fun in it if there are only piles of text laying on top of each other. So, CSS comes to the rescue to make our web pages beautiful. User interface, right? Great! But we human are a cursious kind of creature and we never satisfy. We get bored with static files pretty soon and then JavaScript arrives.
Rethinking the traditional seperation of concerns
The history have decided the way how we used to seperated our concerns - the DOM, CSS and Logic(JavaScript). Again, we are human and laziness is in our nature so we hate repeated tasks. In the software world, we invented a wonderful word to hide our lazy nature and call it "code reusability". Now our traditional way of seperating things up make a lot of senses but if we consider about code resuability, we realize that is not the best way to do it. You may be able to take out some CSS and reuse it which is what the CSS libaries, for example, Bootstrap, are doing. You may also take out some logic and reuse it which is what the JavaScript libaries, for example, JQuery, are doing. (Of course, JQuery can do more than that. I am just focusing on the reusability here.) However, in general, it is not the best. (Easy for us to say it now but it actually takes years and years failure and experience for developers to realize it)
New way of doing it
Let's relate a little to reality, how do we reuse things in real life? If the tires on your vehicle worn out, you don't throw the vehicle away(if you do, please call me first), you just replace them. Similarly, if your battery dies, you simply get a new one. So we break down a big chunk into smaller pieces(In React, they are called Components). Hurray! You just learned the concept behind React. Now let us talk about React. In React, the view and logic are grouped together to build up a Component and the Component can be reused. Indeed, it makes sense that view and logic should be tightly coupled for better code reusability. With that concept, a bunch of smart developers from Facebook or Meta decided to build a framework. Of course that is not the only reason why they developed React. Another obvious reason is to handle the diffrences between browser companies Web APIs since different browsers have their own JavaScript Engine.
Why React?
With enough digging, let us pause and summarize what we have learned and figure out the big "WHY". So why should we use React?
1. React is the trend
React has been tested and approved by many big companies, for example, Meta, Instragram, Microsoft, you name it. Among the most widely used three frameworks (React, Vue, Angular), React still have the most users. This also means React is one of the most demanding skills in front-end development. With a simple search, you will find that 7, 8 out of 10 companies require React as a skill from a front-end developer.
2. React is declarative and state-driven
One of the reason that made React so successful is due to its simplicity. React is declarative instead of imperative. You simply tells React what you want to render and React will handle the rest. Also, React is state-driven which saves you from trying to manage state by yourself.
3. React is faster and more efficient
Thanks to React virtual DOM, we don't have to manually manipulate the real DOM which could have been a real pain. Another benefit by using virtual DOM and React's diff algorithm is performance boost. Instead of updating DOM one by one and triggering restructure of the DOM. React can automatically bundle up some of the actions and make changes to the real DOM only when necessary.
4. React variant can work on other platform
Once we have learnt React, we can pick up React Native and React VR easily. That means we can code for not only browsers but also mobile and even VR. How exciting.
A Peek at React
Enough of history, finally, we can talk about React. Before fully diving into React, let's briefly go over some basic concepts.
What is a Component?
We can think of a component as a state machine. It may contain data, called state and the component will generate HTML via a syntax sugar called JSX. Whenever the maintained data changes, the machine will rerun and generate something different based on the new state.
Class Component vs Function Component
There are two types of Components in React: Class Component and Function Component. Before React 15.8, state only exist in class component so if we want to use state we will have to make it a class component. (We will cover state in the next post. For now, just simply think state as anything that can change in a web page.)
Component from React to React Hooks
With that in mind, let us see how we can create a component.
1. Create React Component with createClass()
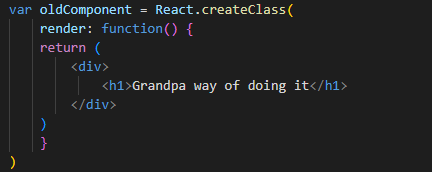
2. Create React Component with extending the React.Component class
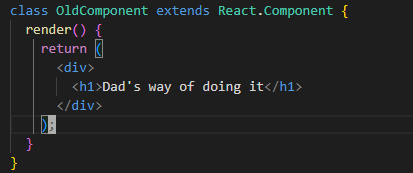
3. Create React Component with hooks
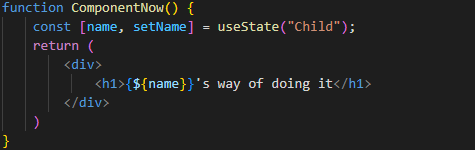
Benefit of using hooks
We have looked at both class component and functional component. We have also looked at the historical way of creating React component and how React Hooks creates component. What is the reason to use React Hooks?
1. Easy to understand
A class component has to extend the React.Component object to get the sweetness of React (capabilities of React like setState() and lifecycle functions). Also, in class component, we have to write constructor and call the super() method to inherite all the functions and properties (Even though there is a work around, you need a deep understanding of JavaScript to not confuse yourself). For event handlers, we have to use bind() in the constructor to access the component instance "this" (Can be solved by using arrow function) Not to mention all the lifecycle methods, when and how to use them. With React hooks, everything becomes cystal clear. Call one function to get the state. Another to manage the side effect and so on. If everyone can understand it, guess what, "LESS BUGS".
2. Better Code Reusability
In class components, because of the lifecycle methods, components are tightly coupled. Tightly coupled also means less reusability. On the other hand, functional components make code reuse much easier. You can even create your own hook to abstract some logic that can be easily transfered to another project.
Diving into details of React
State and Props
State is an object that contain properties that corresponds to the data required by the associated component. When state changes, it triggers re-render of the component which is controlled by React. In class component, we can call the setState() function to trigger a state change. One thing to keep in mind is that state should stay immutable. (Thanks to ES6 syntax, this is not as hard as it sounds)
Another important concept is props. Props is similar to state. Instead of maintaining it locally as state, props are properties that will be passed down to child components of a component. Like state, when props change, a re-render will be triggered on the child components. We can think of props as a shared state with some limitation.
Code Demonstration: Replace class component with React Hook
Now let us try to convert a simple React App which is using class component to one that uses functional component.
1. Change all the class component syntax to functional component syntax
For example, we can make the following changes:
The code below is a typical class component, as we can clearly see it use the "class" keyword and "extends" the React.Component class.
// Class component
class App extends React.Component {...}
JavaScript provides us three ways of declare a function but the two most commonly used ones are:
- Arrow Function
- Function Declaration
In this demo, I will be using arrow function.
2. Get rid of the render() method
In a functional component we don't have the render() method. Instead React will render whatever is returned by the functional component. So we can simply extract the code inside render() method return value and remove the render(). Other code inside the functional component can be moved to the function body.
3. Replace state and setState() by using useState() hook
In a functional component, we don't have access to the state, setState() and other lifecycle methods. The reason being we didn't extend the React.Component class. Therefore, we need to replace them by using the hooks. In this example, the only hook we are going to use is the useState() hook which can be used to substitute the state and setState() method.
4. Modify the instance method
When defining a class in JavaScript using the "class" keyword, since all the methods are properties of the instance or the class if they are static, they have no declaration. However, when we switch to using functional component, we have to declare the type when using arrow functions. A good practise is to use ES6 "const" keyword to declare them as constants.
5. Test and fix other issues
We may run into some other issues after we complete the previous steps. So this is the time to check if we have any bugs and don't forget to open up the console and look for warnings there.
Video Demonstration
If the embedded video is not working, please use the link below to watch it.
Click here to watch the videoConclusion
In this blog, we have looked at the Gensis of React, how we get from where we were to here today. From HTML to CSS, to JavaScript to JavaScript Libraries to front-end frameworks like React. Hopefully, that makes it clear on the very little step of FrontEnd Development. Then, we talked about React and its main concepts: state and props. Finally, we went through a simple demo on how we can refactor our code from using class component to functional component. I hope you've got your time worth reading this blog and learnt something. In my next blog, we will go over some more hooks function. See you all there!
Source Code
https://github.com/NirvanaPhX/React-TodoList